Tools Overview
Tools are specialized functions that enhance the chat engine's capabilities by performing specific tasks, retrieving information, or executing custom logic.
Using Tools in the UI
For users interacting with the chat engine through the UI, tools provide additional capabilities such as:
- Fetching real-time data (e.g., current date, weather updates, events list, etc.)
- Performing calculations (e.g., sum of numbers, conversions)
- Integrating with external services (e.g., Google Calendar, OpenAI API)
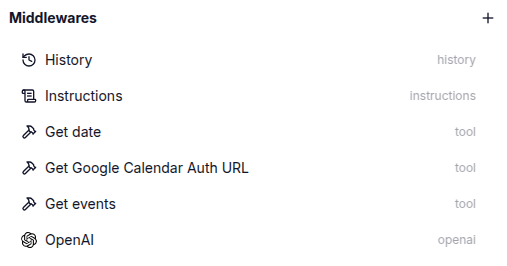
Example: Checking the Current Date
- Type a message like: "What’s today’s date?"
- The chat engine detects the need for a tool and invokes it automatically.
- You receive a response with the current date.
Example: Performing a Calculation
- Type: "What’s 5 + 3?"
- The system uses the
calculateSum
tool to compute the result. - You receive the response: "The sum is 8"
Using Tools in the Chat Engine
Developers can define and register tools within their chat engine instance to enable custom functionalities. Tools are registered with a name, description, parameters, and a method that implements their logic.
Defining and Registering Tools
import { ChatEngineBuilder } from '@indigohive/cogfy-chat-engine'
const app = new ChatEngineBuilder()
.instructions({ instructions: 'You are a helpful assistant that talks like a pirate' })
.tool({
name: 'getDate',
description: 'Get the current date',
method: async (ctx, args) => {
const date = new Date().toISOString()
return { content: date }
}
})
.openai({ model: 'gpt-4o-mini', openai: { apiKey: process.env.OPENAI_API_KEY } })
.build()
;(async function main () {
const result = await app.run({
messages: [
{ role: 'user', content: 'What time is it now in Japan?' }
]
})
console.log(result.messages)
console.log(JSON.stringify(result.trace, null, 2))
})()
Using Predefined Tools in the Chat Engine
import { ChatEngineBuilder, getDate } from '@indigohive/cogfy-chat-engine'
const app = new ChatEngineBuilder()
.instructions({ instructions: 'You are a helpful assistant that talks like a pirate' })
.tool(getDate.tool())
.openai({ model: 'gpt-4o-mini', openai: { apiKey: process.env.OPENAI_API_KEY } })
.build()
;(async function main () {
const result = await app.run({
messages: [
{ role: 'user', content: 'What time is it now in Japan?' }
]
})
console.log(result.messages)
console.log(JSON.stringify(result.trace, null, 2))
})()
Tools with Parameters
Tools can accept parameters to define their behavior and input data. Here’s an example of a tool that calculates the sum of two numbers:
import { ChatEngineBuilder } from '@indigohive/cogfy-chat-engine'
const app = new ChatEngineBuilder()
.instructions({ instructions: 'You are a helpful assistant' })
.tool({
name: 'calculateSum',
description: 'Calculate the sum of two numbers',
parameters: {
type: 'object',
properties: {
a: { type: 'number' },
b: { type: 'number' }
},
required: ['a', 'b']
},
method: async (ctx, args) => {
const sum = args.a + args.b
return { content: `The sum is ${sum}` }
}
})
.build()
;(async function main () {
const result = await app.run({
messages: [
{ role: 'user', content: 'Calculate the sum of 5 and 3' }
]
})
console.log(result.messages)
console.log(JSON.stringify(result.trace, null, 2))
})()
Conclusion
Tools extend the chat engine’s functionality for both end-users and developers. Users can interact with tools via the UI to access real-time data, perform calculations, and integrate services. Developers can register custom tools using the API to provide tailored functionalities. By leveraging tools, you can create a more dynamic and context-aware chat experience.